PrestaShop WhatsApp Order Notifications (Unofficial API)
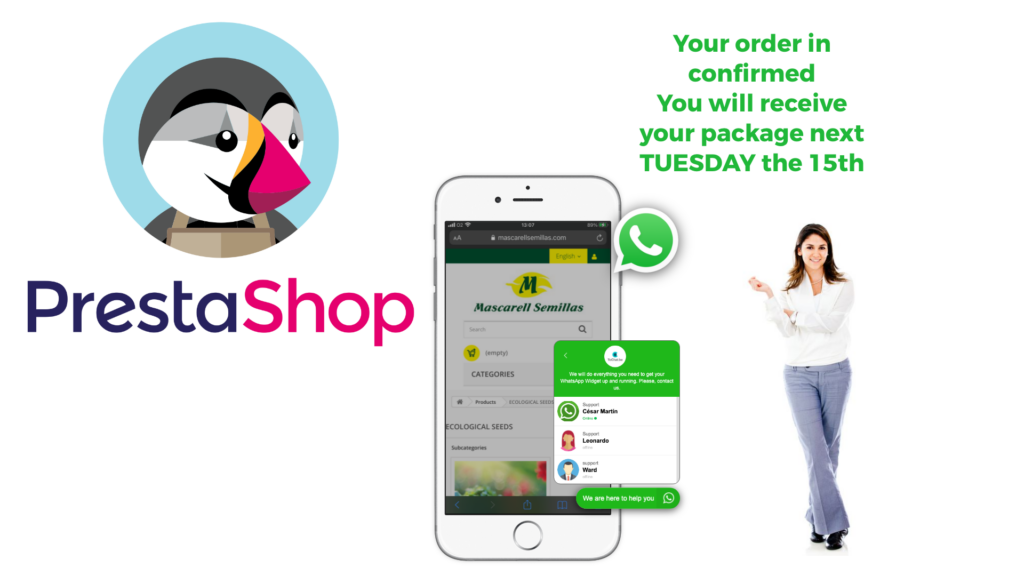
Creating a plugin for PrestaShop to send WhatsApp notifications for order status changes involves several steps. Here’s a detailed guide on how to achieve this:
Table of Contents
1. Create the Module Folder and Main File
Step 1: Create a folder named whatsappnotifications
in the modules/
directory of your PrestaShop installation.
Step 2: Inside this folder, create a file named whatsappnotifications.php
.
2. Main Module File
Add the following code to the whatsappnotifications.php
file:
<?php
if (!defined('_PS_VERSION_')) {
exit;
}
class WhatsappNotifications extends Module
{
public function __construct()
{
$this->name = 'whatsappnotifications';
$this->tab = 'administration';
$this->version = '1.0.0';
$this->author = 'Your Name';
$this->need_instance = 0;
parent::__construct();
$this->displayName = $this->l('WhatsApp Notifications');
$this->description = $this->l('Sends WhatsApp notifications on order status changes.');
}
public function install()
{
return parent::install() &&
$this->registerHook('actionOrderStatusPostUpdate') &&
$this->createConfiguration() &&
$this->createMessageLogsTable();
}
public function uninstall()
{
return parent::uninstall() &&
$this->deleteConfiguration() &&
$this->dropMessageLogsTable();
}
public function getContent()
{
if (Tools::isSubmit('submitWhatsappNotifications')) {
Configuration::updateValue('WHATSAPP_API_TOKEN', Tools::getValue('WHATSAPP_API_TOKEN'));
Configuration::updateValue('WHATSAPP_INSTANCE_ID', Tools::getValue('WHATSAPP_INSTANCE_ID'));
}
$this->context->smarty->assign(array(
'whatsapp_api_token' => Configuration::get('WHATSAPP_API_TOKEN'),
'whatsapp_instance_id' => Configuration::get('WHATSAPP_INSTANCE_ID'),
'form_action' => $_SERVER['REQUEST_URI']
));
return $this->display(__FILE__, 'views/templates/admin/configure.tpl');
}
protected function createConfiguration()
{
Configuration::updateValue('WHATSAPP_API_TOKEN', '');
Configuration::updateValue('WHATSAPP_INSTANCE_ID', '');
return true;
}
protected function deleteConfiguration()
{
Configuration::deleteByName('WHATSAPP_API_TOKEN');
Configuration::deleteByName('WHATSAPP_INSTANCE_ID');
return true;
}
protected function createMessageLogsTable()
{
$sql = 'CREATE TABLE IF NOT EXISTS `'._DB_PREFIX_.'whatsapp_message_logs` (
`id` INT UNSIGNED NOT NULL AUTO_INCREMENT,
`order_id` INT UNSIGNED NOT NULL,
`message` TEXT NOT NULL,
`response` TEXT NOT NULL,
`sent_at` DATETIME NOT NULL,
PRIMARY KEY (`id`)
) ENGINE='._MYSQL_ENGINE_.' DEFAULT CHARSET=utf8;';
return Db::getInstance()->execute($sql);
}
protected function dropMessageLogsTable()
{
$sql = 'DROP TABLE IF EXISTS `'._DB_PREFIX_.'whatsapp_message_logs`';
return Db::getInstance()->execute($sql);
}
public function hookActionOrderStatusPostUpdate($params)
{
$order = new Order($params['id_order']);
$new_status = $params['newOrderStatus']->name;
$jid = '91' . $order->getCustomer()->phone . '@s.whatsapp.net';
$msg = 'Hello ' . $order->getCustomer()->firstname . ', your order #' . $order->id . ' status has changed to ' . $new_status . '.';
$response = $this->sendWhatsappMessage($jid, $msg);
$this->saveMessageLog($order->id, $msg, $response);
}
protected function sendWhatsappMessage($jid, $msg)
{
$token = Configuration::get('WHATSAPP_API_TOKEN');
$instance_id = Configuration::get('WHATSAPP_INSTANCE_ID');
$response = file_get_contents('https://app.send.icu/api/v1/send-text?token=' . urlencode($token) . '&instance_id=' . urlencode($instance_id) . '&jid=' . urlencode($jid) . '&msg=' . urlencode($msg));
return json_decode($response, true);
}
protected function saveMessageLog($order_id, $msg, $response)
{
$sql = 'INSERT INTO `'._DB_PREFIX_.'whatsapp_message_logs` (`order_id`, `message`, `response`, `sent_at`)
VALUES (' . (int)$order_id . ', "' . pSQL($msg) . '", "' . pSQL(json_encode($response)) . '", NOW())';
Db::getInstance()->execute($sql);
}
}
3. Configuration Template
Create a views/templates/admin/
directory inside the whatsappnotifications
folder and add a file named configure.tpl
:
<form action="{$form_action}" method="post">
<label for="whatsapp_api_token">API Token</label>
<input type="text" id="whatsapp_api_token" name="WHATSAPP_API_TOKEN" value="{$whatsapp_api_token}">
<br>
<label for="whatsapp_instance_id">Instance ID</label>
<input type="text" id="whatsapp_instance_id" name="WHATSAPP_INSTANCE_ID" value="{$whatsapp_instance_id}">
<br>
<input type="submit" name="submitWhatsappNotifications" value="Save">
</form>
4. Activating the Module
- Upload the Module: Upload the
whatsappnotifications
folder to themodules/
directory of your PrestaShop installation. - Install the Module: Go to the PrestaShop admin dashboard, navigate to “Modules” -> “Module Manager,” find “WhatsApp Notifications,” and click “Install.”
- Configure the Module: After installation, click “Configure” to enter your WhatsApp API token and instance ID.
By following these steps, you will create a PrestaShop module that sends WhatsApp notifications to customers when their order status changes. The module includes configuration settings for the API token and instance ID and logs the sent messages.